Read data from MySql database from Wamp server and display in Android Client
Step1: create a php file to display all products in JSON Format
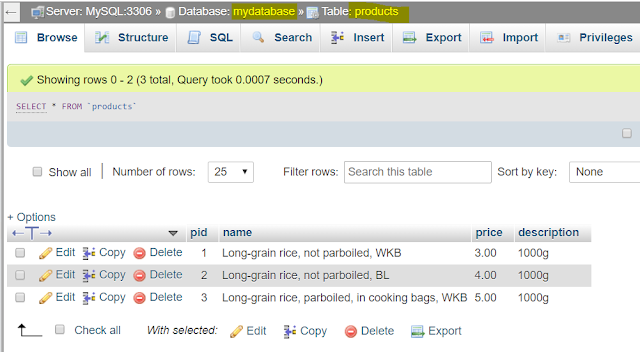
http://mdad.atspace.cc/products/get_all_productsJson.php
We expect to see data displayed in JSON format
{"products":[{"pid":"1","name":"Long-grain rice, not parboiled, WKB","price":"3.00"},{"pid":"2","name":"Long-grain rice, not parboiled, BL","price":"4.00"},{"pid":"3","name":"Long-grain rice, parboiled, in cooking bags, WKB","price":"5.00"}],"success":1}
We can use a JSON formatter to see the data in more organised manner
Copy the JSON data and paste it in the URL below
https://jsonformatter.org/
{
"products": [
{
"pid": "1",
"name": "Long-grain rice, not parboiled, WKB",
"price": "3.00"
},
{
"pid": "2",
"name": "Long-grain rice, not parboiled, BL",
"price": "4.00"
},
{
"pid": "3",
"name": "Long-grain rice, parboiled, in cooking bags, WKB",
"price": "5.00"
}
],
"success": 1
}
Step 4: Edit AndroidManifest.xml
android:usesCleartextTraffic : Indicates whether the app intends to use cleartext network traffic, such as cleartext HTTP. The default value for apps that target API level 27 or lower is
Step 7: We will be using Volley HTTP library that makes networking for Android apps easier and most importantly, faster.
Step 8: Modify MainActivity.java. Copy and paste the codes below:
The line below needs to be changed according to your ip address
OR If you have hosted it in atspace.com then it is even easier for you to test with atspace server without running the Wamp Server
For my case, I test it with http://mdad.atspace.cc/products
and I do not need to start wamp sever and can test it on my phone anytime and anywhere.
public static String ipBaseAddress = "http://mdad.atspace.cc/products";
Step 9: Modify AllProductsActivity.java. Copy and paste the codes below:
Step 10: Now you are ready to Run the App.
Step 11: To add a ProgressDialog bar to the Activity
https://abhiandroid.com/ui/progressdialog
Step11A: Add a class variable
The codes should be added as shown below:
Step1: create a php file to display all products in JSON Format
Let’s name it as get_all_productsJson.php
<?php /* * Following code will list all the products */ // include db connect class require_once __DIR__ . '/db_connect.php'; // connecting to db $db= new DB_CONNECT(); $db->connect(); // get all products from products table $sqlCommand="SELECT *FROM products"; $result =mysqli_query($db->myconn, "$sqlCommand"); // check for empty result if (mysqli_num_rows($result) > 0) { // looping through all results // products node $response["products"] = array(); while ($row = mysqli_fetch_array($result)) { // temporary array $product $product = array(); $product["pid"] = $row["pid"]; $product["name"] = $row["name"]; $product["price"] = $row["price"]; // push single product into final response array array_push($response["products"], $product); } // success $response["success"] = 1; // echoing JSON response echo json_encode($response); } else { // no products found $response["success"] = 0; $response["message"] = "No products found"; // echo no users JSON echo json_encode($response); } ?>
Step 2: Start Wamp server and test it at the internet browser.
Assume you have setup your database as follows:
http://mdad.atspace.cc/products/get_all_productsJson.php
We expect to see data displayed in JSON format
{"products":[{"pid":"1","name":"Long-grain rice, not parboiled, WKB","price":"3.00"},{"pid":"2","name":"Long-grain rice, not parboiled, BL","price":"4.00"},{"pid":"3","name":"Long-grain rice, parboiled, in cooking bags, WKB","price":"5.00"}],"success":1}
We can use a JSON formatter to see the data in more organised manner
Copy the JSON data and paste it in the URL below
https://jsonformatter.org/
{
"products": [
{
"pid": "1",
"name": "Long-grain rice, not parboiled, WKB",
"price": "3.00"
},
{
"pid": "2",
"name": "Long-grain rice, not parboiled, BL",
"price": "4.00"
},
{
"pid": "3",
"name": "Long-grain rice, parboiled, in cooking bags, WKB",
"price": "5.00"
}
],
"success": 1
}
Step 3: Now, we would like create an android app to load data from Mysql database via get_all_productsJson.php. Let’s begin with creating an Android Project
Create a main activity with 2 Buttons
For a quick start, you may copy the XML codes below and paste on the activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" android:gravity="center_horizontal"> <!-- Sample Dashboard screen with Two buttons --> <!-- Button to view all products screen --> <Button android:id="@+id/btnViewProducts" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="View Products" android:layout_marginTop="25dip"/> <!-- Button to create a new product screen --> <Button android:id="@+id/btnCreateProduct" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Add New Products" android:layout_marginTop="25dip"/> </LinearLayout>
Step 4: Edit AndroidManifest.xml
a. We need read data from MySql database via the Internet so we need to set the permission.
b. Edit AndroidManifest.xml. Add use-permission tag with android.permission.INTERNET
as shown below:
c. put this line in application tag in manifest file as shown below:
c. put this line in application tag in manifest file as shown below:
android:usesCleartextTraffic="true"
android:usesCleartextTraffic : Indicates whether the app intends to use cleartext network traffic, such as cleartext HTTP. The default value for apps that target API level 27 or lower is
"true"
. Apps that target API level 28 or higher default to "false"
.<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="networkclient.mdad.sql_databaseexample"> <!-- Internet Permissions --> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:usesCleartextTraffic="true" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Step 5: Create another activity called AllProductsActivity
Right clicked on app>new>Activity>Empty Activity. Name it as AllProductsActivity.
Copy and paste the codes below for activity_all_products.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical"> <!-- Main ListView Always give id value as list(@android:id/list) --> <ListView android:id="@android:id/list" android:layout_width="fill_parent" android:layout_height="wrap_content"/> </LinearLayout>
Step 6: Create a list_item.xml to list the items
Copy and paste the codes below for list_item.xml .
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical" > <!-- Product id (pid) - will be HIDDEN - used to pass to other activity --> <TextView android:id="@+id/pid" android:layout_width="fill_parent" android:layout_height="wrap_content" android:visibility="gone" /> <!-- Name Label --> <TextView android:id="@+id/name" android:layout_width="fill_parent" android:layout_height="wrap_content" android:paddingTop="6dip" android:paddingLeft="6dip" android:textSize="17dip" android:textStyle="bold" /> </LinearLayout>
Step 7: We will be using Volley HTTP library that makes networking for Android apps easier and most importantly, faster.
Goto Grade Scripts> build.gradle(Module: app)
implementation 'com.android.volley:volley:1.1.1'
Add the above line as shown below:
Click on Sync Now as show in the above picture
Step 8: Modify MainActivity.java. Copy and paste the codes below:
The line below needs to be changed according to your ip address
public static String ipBaseAddress = "http://192.168.0.111:81/products";
Find your IP address using windows>cmd>ipconfig
For example if your IP address is IPv4 Address. . . . . . . . . . . : 192.168.0.111:81
http://192.168.0.111:81/products
OR If you have hosted it in atspace.com then it is even easier for you to test with atspace server without running the Wamp Server
For my case, I test it with http://mdad.atspace.cc/products
and I do not need to start wamp sever and can test it on my phone anytime and anywhere.
public static String ipBaseAddress = "http://mdad.atspace.cc/products";
package networkclient.mdad.sql_databaseexample; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.content.Intent; import android.util.Log; import android.view.View; import android.widget.Button; public class MainActivity extends AppCompatActivity { //check your IP address and in my case i use port 81 //public static String ipAddress = "http://192.168.0.111:81/products"; //Or you can use your own public domain address hosted at atspace.com //In my case, it is http://mdad.atspace.cc/products public static String ipBaseAddress = "http://192.168.0.111:81/products"; Button btnViewProducts; Button btnNewProduct; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // MainActivity.ipAddress =getApplicationContext().getResources().getString(R.string.ipAddress); Log.i("---Ip address", ipBaseAddress); // Buttons btnViewProducts = (Button) findViewById(R.id.btnViewProducts); btnNewProduct = (Button) findViewById(R.id.btnCreateProduct); // view products click event btnViewProducts.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // Launching All products Activity Intent i = new Intent(getApplicationContext(), AllProductsActivity.class); startActivity(i); } }); // view products click event btnNewProduct.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // Launching create new product activity Intent i = new Intent(getApplicationContext(), NewProductActivity.class); startActivity(i); } }); } }
Step 9: Modify AllProductsActivity.java.
package networkclient.mdad.sql_databaseexample; import android.os.Bundle; import java.util.ArrayList; import java.util.HashMap; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import android.app.ListActivity; import android.content.Intent; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ListAdapter; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.TextView; import com.android.volley.Request; import com.android.volley.RequestQueue; import com.android.volley.Response; import com.android.volley.VolleyError; import com.android.volley.toolbox.JsonObjectRequest; import com.android.volley.toolbox.Volley; public class AllProductsActivity extends ListActivity { ArrayList<HashMap<String, String>> productsList; // url to get all products list private static String url_all_products = MainActivity.ipBaseAddress+"/get_all_productsJson.php"; // JSON Node names private static final String TAG_SUCCESS = "success"; private static final String TAG_PRODUCTS = "products"; private static final String TAG_PID = "pid"; private static final String TAG_NAME = "name"; // products JSONArray JSONArray products = null; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_all_products); // Log.i("------url_all_products",url_all_products); // Hashmap for ListView productsList = new ArrayList<HashMap<String, String>>(); // Loading products in Background Thread postData(url_all_products,null ); // Get listview from list_items.xml ListView lv = getListView(); // on seleting single product // launching Edit Product Screen lv.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // getting values from selected ListItem // Starting new intent // sending pid to next activity
// starting new activity and expecting some response back } }); } // Response from Edit Product Activity @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); // if result code 100 means Continue //https://www.w3.org/Protocols/rfc2616/rfc2616-sec10.html if (resultCode == 100) { // if result code 100 is received // means user edited/deleted product // reload this screen again Intent intent = getIntent(); finish(); startActivity(intent); } } public void postData(String url, final JSONObject json){ RequestQueue requestQueue = Volley.newRequestQueue(this); JsonObjectRequest json_obj_req = new JsonObjectRequest( Request.Method.POST, url, json, new Response.Listener<JSONObject>() { @Override public void onResponse(JSONObject response) { checkResponse(response, json); // String alert_message; // alert_message = response.toString(); // showAlertDialogue("Response", alert_message); } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { error.printStackTrace(); // String alert_message; // alert_message = error.toString(); // showAlertDialogue("Error", alert_message); } }); requestQueue.add(json_obj_req); } private void checkResponse(JSONObject response, JSONObject creds){ try { if(response.getInt(TAG_SUCCESS)==1){ // products found // Getting Array of Products products = response.getJSONArray(TAG_PRODUCTS); // looping through All Products for (int i = 0; i < products.length(); i++) { JSONObject c = products.getJSONObject(i); // Storing each json item in variable String id = c.getString(TAG_PID); String name = c.getString(TAG_NAME); // creating new HashMap HashMap<String, String> map = new HashMap<String, String>(); // adding each child node to HashMap key => value map.put(TAG_PID, id); map.put(TAG_NAME, name); // adding HashList to ArrayList productsList.add(map); } /** * Updating parsed JSON data into ListView * */ ListAdapter adapter = new SimpleAdapter( AllProductsActivity.this, productsList, R.layout.list_item, new String[] { TAG_PID, TAG_NAME}, new int[] { R.id.pid, R.id.name }); // updating listview setListAdapter(adapter); } else{ } } catch (JSONException e) { e.printStackTrace(); } } } //end of AllProductsActivity class
Step 10: Now you are ready to Run the App.
Remember to change your IP address at the MainActivity.java and start WAMP server
public static String ipBaseAddress = "http://192.168.0.111/products";
OR If you have hosted it in atspace.com then it is even easier for you to test with atspace server without running the Wamp Server
For my case, I test it with http://mdad.atspace.cc/products
and I do not need to start wamp sever and can test it on my phone anytime and anywhere.
public static String ipBaseAddress = "http://mdad.atspace.cc/products";
OR If you have hosted it in atspace.com then it is even easier for you to test with atspace server without running the Wamp Server
For my case, I test it with http://mdad.atspace.cc/products
and I do not need to start wamp sever and can test it on my phone anytime and anywhere.
public static String ipBaseAddress = "http://mdad.atspace.cc/products";
Step 11: To add a ProgressDialog bar to the Activity
https://abhiandroid.com/ui/progressdialog
Step11A: Add a class variable
// Progress Dialog private ProgressDialog pDialog;
Step11B: Add the following codes to Load the Progress DialogUse alternate+Enter to import the statement import android.app.ProgressDialog;
pDialog = new ProgressDialog(this); pDialog.setMessage("Loading product ..."); pDialog.setIndeterminate(false); pDialog.setCancelable(true); pDialog.show();
The codes should be added as shown below:
Step11C: Add one line of code to Dismiss the Progress Dialog
pDialog.dismiss();
The code should be added as shown below:
No comments:
Post a Comment
Note: only a member of this blog may post a comment.