Objectives
- To add a new Page (Activity) to calculate BMI
- To make use of EditText, TextView and Button
- To use methods like getText(), Double.parseDouble.
//move from MainActivity to Main2Activity
Intent intent= new Intent(MainActivity.this,Main2Activity.class); startActivity(intent);
etWeight.getText().toString(); //get the text from EditText and convert to string
Double.parseDouble("1.72" ); //convert String "1.72" to double 1.72
//#1 Declare EditText EditText etWeight, etHeight; //#2 bind java object to xml etWeight = (EditText) findViewById(R.id.editText1); //#3 get text, the weight and height from the user and //convert it to double/decimal numbers double weight = Double.parseDouble(etWeight.getText().toString());
Step1: Create a new Empty Activity. U need to rebuild project if encounter error.
Step2: Drag in a LinearLayout (Vertical).
Drag in a TextView , EditText and Button as shown below
Step3:
Change the text of TextView
For EditText Change the InputType to number so that it can only accept numbers and not text
Step 5: Add the highlighted codes to onClick method of MainActivity.java as shown below
button.setOnClickListener(new View.OnClickListener(){ public void onClick(View v){ //Add a new page here in this case Main2Activity //move from MainActivity to Main2Activity Intent intent= new Intent(MainActivity.this,Main2Activity.class); startActivity(intent); ..... .... ....
Use Intellisense , Alt+Enter to import class whenever necessary
When u run the app you expect to see the next Activity Main2Activity being launched when user click on submit button of mainAcitivity
//move from MainActivity to Main2Activity
Intent intent= new Intent(MainActivity.this,Main2Activity.class); startActivity(intent);Step 6: Add the highlighted codes to Main2Activity.java as shown below:
public class Main2Activity extends AppCompatActivity { //#1 Declare EditText, Button and TextView EditText etWeight, etHeight; Button button; TextView tvBMI;
Add the highlighted codes inside the onCreate method as shown below
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main2); //#2 bind these 3 java objects to xml etWeight = (EditText) findViewById(R.id.editText1); etHeight = (EditText) findViewById(R.id.editText2); tvBMI = (TextView) findViewById(R.id.textView3); button = (Button)findViewById(R.id.button1); //#3 Button click Listener button.setOnClickListener(new View.OnClickListener(){ public void onClick(View v){
} });
Add the highlighted codes inside the onClick method
//#4 get text, the weight and height from the user //and convert it to double/decimal numbers double weight = Double.parseDouble(etWeight.getText().toString()); double height = Double.parseDouble(etHeight.getText().toString()); //#5 Calculate BMI double bmi = weight/height/height; //#6 show the BMI results tvBMI.setText("Your BMI is "+bmi);
Run the app, put in some values and check if the BMI value is calculated and displayed correctly
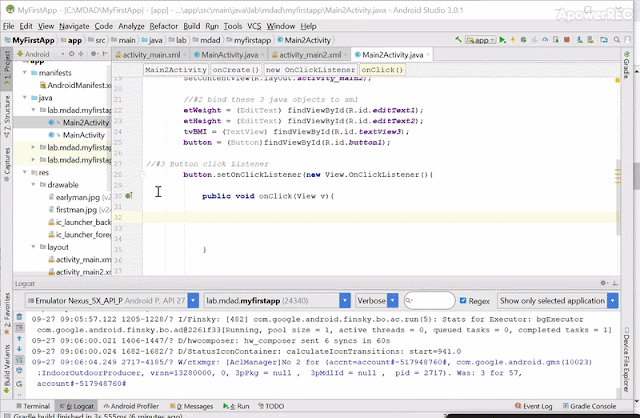
Hint: If you could not enter decimal point then you would need to change the InputType of the EditText in the XML layout. This step is not shown in the video intentionally.
Demo and explanation of codes
No comments:
Post a Comment
Note: only a member of this blog may post a comment.